- Sloth Bytes
- Posts
- 🦥 Unit Testing: Test Doubles
🦥 Unit Testing: Test Doubles
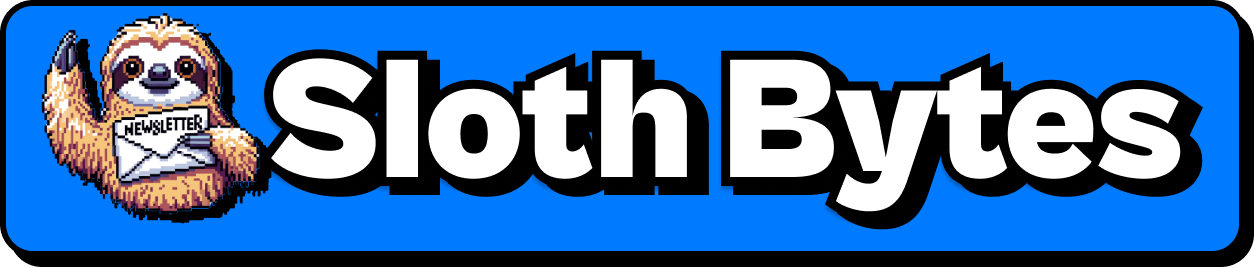
Hello friends!
Welcome to this week’s Sloth Bytes!
I hope you had a horrible week. 😉

Learn AI in 5 minutes a day
This is the easiest way for a busy person wanting to learn AI in as little time as possible:
Sign up for The Rundown AI newsletter
They send you 5-minute email updates on the latest AI news and how to use it
You learn how to become 2x more productive by leveraging AI

Sloths prefer to eat leaves from certain trees.
A sloth will rotate among approximately 7 to 12 favorite feeding trees.
It’s a a strategy that prevents them from overeating any one kind of leaf.

Unit Testing: Test Doubles

I’ve recently been studying some programming concepts…
(wow programming YouTuber needs to study programming)
I was specifically researching about unit tests because I don’t test my code enough and never really learned how.
I came across these interesting terms for unit testing:
Mocking, Stubbing, and Spying.
What’s interesting about these concepts is they’re all strategies for what’s called:
Test Doubles.
What the flip is a Test Double?
Test doubles are objects that look and act as components in your app but they're created in your test to provide a specific behavior or data.
Why do we need Test Doubles?
Test doubles are important because we have to test code that depends on other components.
We have to test our code on:
APIs that aren't ready
Databases that are slow
Services that cost money
Code that's complex
Random behavior
So we create test doubles that mimic those dependencies in order to isolate the code we want to test without it affecting other components.
Other benefits are
Faster tests
Simplified tests
More control
Types of Test Doubles
This is where the 3 strategies show up:
1. Mocks
Provide responses but they also track the interactions.
They’re set up with expectations about which methods should be called and with what arguments.
Allow you to verify that the system interacts with its dependencies in a specific way.
// Fake expects specific method calls and arguments
const fakeDatabase = jest.fn();
fakeDatabase.save
.expect()
.withArgs(userData)
.toBeCalledTimes(1);
2. Stubs
Provide hardcoded responses to calls made during tests.
Are used to simulate dependencies by returning fixed data.
Do not record how they are called; their focus is solely on providing the necessary inputs for the test.
Stubs are essentially mocks without expectations. The goal here is not to track the interactions. Only just the responses/outputs.
// Real payment processor
function processPayment(amount) {
// Complex payment logic that's hard to test and slow.
}
// Stub version (simplified version to make it easier to test with)
const paymentStub = {
processPayment: () => true
};
3. Spies
Doesn’t create a double, instead it “spies” on the real function.
Spies capture details such as how many times a function was called, with which arguments, and sometimes even the return values.
They allow you to later assert that certain interactions occurred (or did not occur).
Unlike Mocks or Stubs, Spies mainly observe to verify that responses/interactions are working properly
// Spy tracks calls but keeps original behavior
const spy = jest.spyOn(realDatabase, 'save');
// service is the business logic layer that uses the database
// We want to spy on the interaction between service and database.
await service.createUser(userData);
// We expect that the interaction is successful and userData is now in the database.
expect(spy).toHaveBeenCalledWith(userData);



Thank you to everyone who submitted 😃
RelyingEarth87, porrrq, E-Sieben, ravener, in1yan, Raufirzaman, paarthjuneja, GabrielDornelas, and tobiaoy.
Let’s have an easier week.
Phone Number Formatting
Create a function that takes a list of 10 numbers (between 0 and 9) and returns a string of those numbers formatted as a phone number (e.g. (555) 555-5555).
Examples
format_phone_number([1, 2, 3, 4, 5, 6, 7, 8, 9, 0])
output = "(123) 456-7890"
format_phone_number([5, 1, 9, 5, 5, 5, 4, 4, 6, 8])
output = "(519) 555-4468"
format_phone_number([3, 4, 5, 5, 0, 1, 2, 5, 2, 7])
output = "(345) 501-2527"
Notes
Don't forget the space after the closing parenthesis.
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!
If you want to be mentioned here, I’d prefer if you sent a GitHub link or Replit!

2 Videos might come out this week
Yeah you heard me, double upload, but uh that’s because it’s almost been a month since the last upload (whoops.)
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
Reply