- Sloth Bytes
- Posts
- 🦥 Static Typing vs Dynamic Typing
🦥 Static Typing vs Dynamic Typing
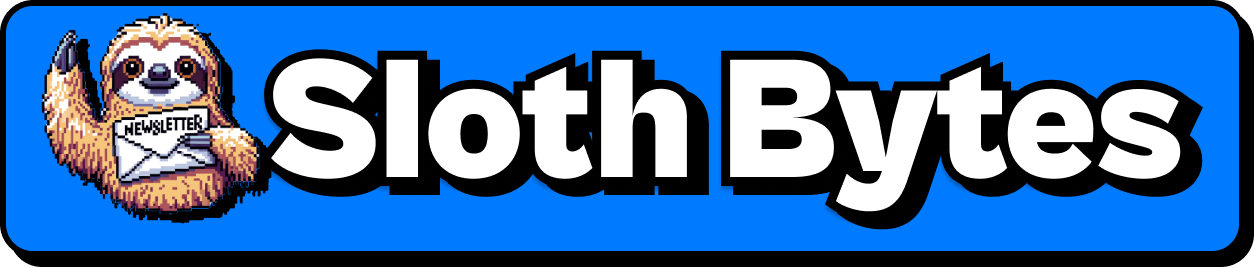
Hello friends!
Welcome to this week’s Sloth Bytes. I hope you had a great week!
As always, if you have any suggestions or if I got something wrong let me know!

Sloths have a lot of bugs in their fur
Just one sloth can have up to 950 moths and beetles living in its fur. Don’t worry! These aren’t parasites. When one animal lives on another animal in this way, it’s called symbiosis.

Static vs Dynamic Typing

What is this?
Static Typing: Types are checked at compile time
int your_iq = 0;
Dynamic Typing: Types are checked at runtime
your_iq = 0
Key Differences
Type Declaration:
Static: Variables declared with specific types
Dynamic: Variables can hold any type
Error Detection:
Static: Many errors caught before running
Dynamic: Errors often surface during execution
Performance:
Static: Generally faster execution
Dynamic: More flexible, potentially slower
Code Flexibility:
Static: Less flexible, more rigid
Dynamic: More flexible, easier to change
Examples of SOME Statically Typed Languages
Java
C++
Rust
Swift
Kotlin
Scala
Haskell
Examples of SOME Dynamically Typed Languages
Python
JavaScript
Ruby
PHP
Lua
Pros and Cons
Static Typing✅ Earlier error detection ✅ Better performance ✅ Clear documentation ❌ More verbose code ❌ Less flexibility | Dynamic Typing✅ Faster development ✅ More flexible code ✅ Easier to work with ❌ Runtime errors more common ❌ Can be harder to understand |
Which to Choose?
From my experience, this is what I think. I could be wrong (I probably am)
Large, complex projects: Static typing
Making something fast: Dynamic might be better
Other alternatives
Gradual typing: Add type hints to dynamic languages (e.g., Python with type hints)
TypeScript: Adds static typing to JavaScript
Why Should I Care?
Choose the right language for your project
Write more robust and maintainable code
Anticipate and prevent certain types of bugs
Remember: Both systems have their place. The best choice depends on your project needs, team skills, and development goals. Many modern languages are adopting features from both worlds!

Stacks and Queues

What are Stacks and Queues?
Stack: Last-In-First-Out (LIFO) data structure. The last element added is the first that goes out. Think of it like a stack of pancakes. Normal people start at the top of the stack and make their way to the bottom.
Queue: First-In-First-Out (FIFO) data structure. The First item that gets added is the first that goes out. Think of it as a line/waiting queue, the first person there gets to leave.
Stack Operations and Time Complexity:
push(item): Add item to top - O(1)
pop(): Remove and return top item - O(1)
peek(): View top item without removing - O(1)
isEmpty(): Check if stack is empty - O(1)
Queue Operations and Time Complexity::
enqueue(item): Add item to back - O(1)
dequeue(): Remove and return front item - O(1)
front(): View front item without removing - O(1)
isEmpty(): Check if queue is empty - O(1)
Stack Implementation (Python):
# Stack implementation
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def peek(self):
return self.items[-1]
def isEmpty(self):
return len(self.items) == 0
Queue Implementation (Python):
# Queue implementation
class Queue:
def __init__(self):
#if you use the deque library you could do
#self.items = deque()
self.items = []
def enqueue(self, item):
self.items.append(item)
def dequeue(self):
#if you use the deque library you could do
#return self.items.popleft()
return self.items.pop(0)
def front(self):
return self.items[0]
def isEmpty(self):
return len(self.items) == 0
Key Points to Remember
Understand the difference between LIFO and FIFO
Know how to implement both structures
Be familiar with time complexities of operations
Practice problems involving multiple stacks/queues
Understand when to use stacks vs queues in problem-solving
Why This Matters
Fundamental to many algorithms and data structures
Often used in depth-first search (stack) and breadth-first search (queue)
Common in system design (e.g., task scheduling, undo functionality)
Remember: Practice implementing these structures from scratch and solving related problems. Understanding when and how to use stacks and queues can greatly improve your problem-solving skills in interviews!

Learn AI in 5 Minutes a Day
AI Tool Report is one of the fastest-growing and most respected newsletters in the world, with over 550,000 readers from companies like OpenAI, Nvidia, Meta, Microsoft, and more.
Our research team spends hundreds of hours a week summarizing the latest news, and finding you the best opportunities to save time and earn more using AI.




Thank you to everyone who submitted last week!
tobiaoy, xitsjacob, ShiSui97x, afiqzudinhad, QueenlyHeart, ravener, RelyingEarth87, ddat888 and last but not least…

Alright, let’s do some stack/queue practice
Implement a Stack using Queues
Implement a Stack using only two queues. The implemented stack should support all the functions of a normal stack (push, top, pop, and empty).
Implement the MyStack class:
void push(int x) Pushes element x to the top of the stack.
int pop() Removes the element on the top of the stack and returns it.
int top() Returns the element on the top of the stack.
boolean empty() Returns true if the stack is empty, false otherwise.
Example:
Input
["MyStack", "push", "push", "top", "pop", "empty"]
[[], [1], [2], [], [], []]
Output
[null, null, null, 2, 2, false]
Explanation
MyStack myStack = new MyStack();
myStack.push(1);
myStack.push(2);
myStack.top(); // return 2
myStack.pop(); // return 2
myStack.empty(); // return False
Follow-up: Can you implement the stack using only one queue?
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!

SLOTH WHERE NEW VID 😡
uhhhh…. I’m working on it (I swear). It’s going to be about some tools I like to use for programming/productivity, I think most of you will like it!
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply