- Sloth Bytes
- Posts
- š¦„Recursion For Dummies
š¦„Recursion For Dummies
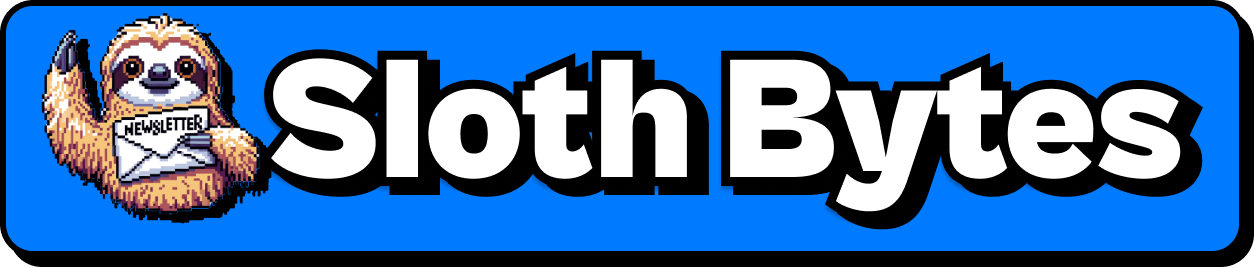

Hello friends!
Welcome to this weekās Sloth Bytes!
I hope you had a great week.

Never explain another bug with Jam AI.
Say goodbye to vague bug reports.
Jam turns any bug into a complete technical report in one click.
Records issue & auto-generates technical description
Creates step-by-step reproduction guide
Captures all debug essentials (console, network, environment)
Integrates with Jira, GitHub, Linear & more
Join 140K+ developers who fixed the bug reporting bottleneck.
One click. Perfect bug reports. Every time.

Sloths have small brains.
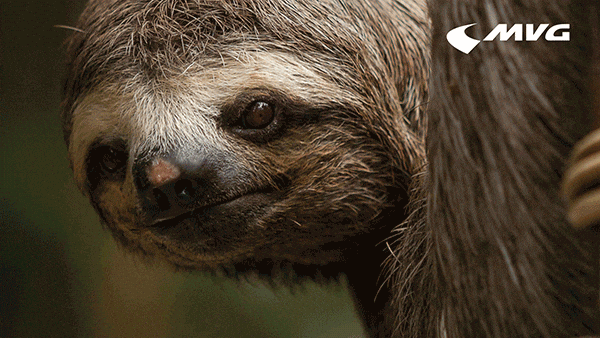
Gif by MVG on Giphy
HOWEVER, scientists are now realizing that this actually doesnāt relate to intelligence at all.
The brains of sloths might be small but they are very much focused on the specific skills that they need for survival.

Recursion: It's Not as Scary as You Think

Ever been told "just use recursion" and felt your brain melt? Let me simplify this concept with some simple examples.
What is Recursion?
Think of it like those Russian nesting dolls:
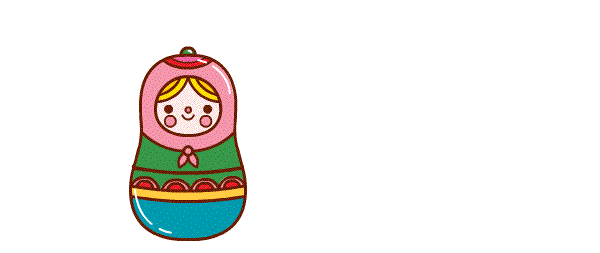
Gif by cecymeade on Giphy
Each doll contains a smaller version of itself
Until you reach the smallest doll
That's it. That's recursion.
For programming recursion is a function that calls itself until it shouldn't.
Why Use It?
Perfect for problems that:
Have repeated smaller sub-problems
Follow a pattern
Deal with trees or nested structures
Need to traverse directories
The 2 Steps of Recursion
Every recursive function needs:
A base case (when to stop)
A recursive case (when to continue)
That's all! Itās that simple.
Example
Calculating a factorial (5! = 5 Ć 4 Ć 3 Ć 2 Ć 1)
// ā Without recursion (iterative)
function factorial(n) {
let result = 1;
for(let i = n; i > 0; i--) {
result *= i;
}
return result;
}
// ā
With recursion (cleaner!)
function factorial(n) {
// Base case: stop at 1
if (n === 1) return 1;
// Recursive case: n * factorial of (n-1)
return n * factorial(n - 1);
}
Whatās happening?
factorial(5) breaks down like this:
factorial(5)
ā 5 * factorial(4)
ā 4 * factorial(3)
ā 3 * factorial(2)
ā 2 * factorial(1)
ā 1 // We hit our Base case! Let's go back and add it up.
ā 2 * 1 = 2 // 2 * factorial(1), factorial(1) = 1
ā 3 * 2 = 6 // 3 * factorial(2), factorial(2) = 2
ā 4 * 6 = 24 // 4 * factorial(3), factorial(3) = 6
ā 5 * 24 = 120 // 5 * factorial(4), factorial(4) = 24
Common Use Cases
Directory traversal
File systems
JSON structures
DOM elements
Tree operations
Binary trees
File explorers
Menu structures
Divide and conquer
Sorting algorithms
Search algorithms
Mathematical calculations
When Not to Use It
The problem is simple
You need maximum performance
Stack space is limited
The depth is unknown
Quick Tips
ALWAYS have a base case (youāll be stuck in an infinite loop if you donāt)
Move towards the base case
Keep it simple
Test with small inputs first
Remember
It's just a function calling itself
Always have a way to stop
Always think of recursion as "breaking it down"
Visualize the steps
Practice makes perfect



Thank you to everyone who submitted š
GabrielDornelas, E-Sieben, levi-manoel, TheTigerPython, RelyingEarth87, SDKwapis, porrrq, and nhillemann.
Remove the Computer Virus
Your computer might have been infected by a virus! Create a function that finds the viruses in files
and removes them from your computer.
Examples
remove_virus("PC Files: spotifysetup.exe, virus.exe, dog.jpg")
output = "PC Files: spotifysetup.exe, dog.jpg"
remove_virus("PC Files: antivirus.exe, cat.pdf, lethalmalware.exe, dangerousvirus.exe ")
output = "PC Files: antivirus.exe, cat.pdf"
remove_virus("PC Files: notvirus.exe, funnycat.gif")
output = "PC Files: notvirus.exe, funnycat.gif")
Notes
Bad files will contain "virus" or "malware", but "antivirus" and "notvirus" will not be viruses.
Return
"PC Files: Empty"
if there are no files left on the computer.
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if youāre on the web version leave a comment!
If you want to be mentioned here, Iād prefer if you sent a GitHub link or Replit!

Working on the next video and uh yeah thatās about it.
Thatās all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
Reply