- Sloth Bytes
- Posts
- 🦥Introduction To React
🦥Introduction To React
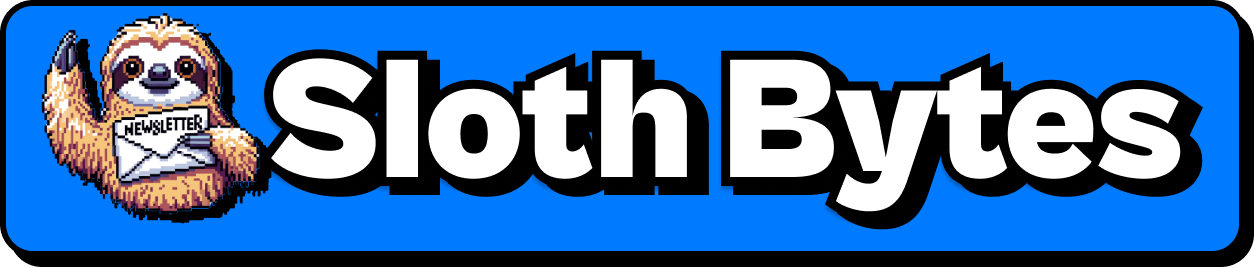
Hello friends!
Welcome to this week’s Sloth Bytes.
I hope you had an amazing week.

Writer RAG tool: build production-ready RAG apps in minutes
Writer RAG Tool: build production-ready RAG apps in minutes with simple API calls.
Knowledge Graph integration for intelligent data retrieval and AI-powered interactions.
Streamlined full-stack platform eliminates complex setups for scalable, accurate AI workflows.

Sloths need to stay warm to digest their food
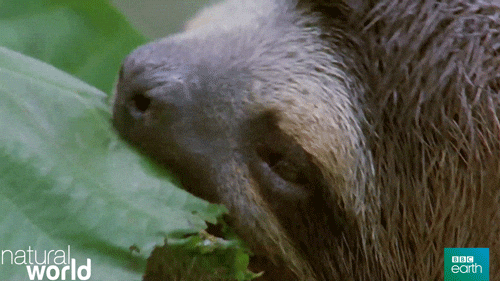
Gif by bbcearth on Giphy
If they get too cold, sloths can die of starvation even on a full stomach. This is because the bacteria which breaks down the leaves will die if a sloth’s body temperature falls too low, leaving undigested leaves in their stomach which are unable to pass along or be thrown up.

Introduction to React

React has become the most popular JavaScript library for building user interfaces, But what makes it special?
Why React's Popularity Keeps Growing
Component-Based: Build reusable UI pieces like Lego blocks
Virtual DOM: Automatically optimizes updates for better performance
Massive Ecosystem: A LOT of npm packages to solve common problems
Strong Community: Lots of resources for React.
Battle-Tested: Used by Facebook, Instagram, Netflix, and Airbnb
Great Developer Tools: React DevTools make debugging easier.
Cross-Platform: Build mobile apps with React Native
Future-Proof: Regular updates and active development by Meta
How React Makes Development Easier
1. Declarative vs Imperative
Instead of telling the computer HOW to do something (imperative), React lets you describe WHAT you want (declarative).
2. Components Make Code Reusable
Think of components as custom HTML elements. Here's a simple example:
Vanilla JavaScript:
// Creating multiple cards means repeating this code
const card1 = document.createElement('div');
card1.className = 'card';
const title1 = document.createElement('h2');
title1.textContent = 'Card 1';
card1.appendChild(title1);
document.body.appendChild(card1);
React:
// Create once, use anywhere
function Card({ title }) {
return (
<div className="card">
<h2>{title}</h2>
</div>
);
}
// Use it multiple times
function App() {
return (
<div>
<Card title="Card 1" />
<Card title="Card 2" />
<Card title="Card 3" />
</div>
);
}
3. State Management Made Simple
Let's look at a more complex example with a todo list:
Vanilla JavaScript:
// HTML
<div>
<input type="text" id="todoInput">
<button onclick="addTodo()">Add</button>
<ul id="todoList"></ul>
</div>
// JavaScript
let todos = [];
function addTodo() {
const input = document.getElementById('todoInput');
todos.push(input.value);
input.value = '';
updateTodoList();
}
function updateTodoList() {
const list = document.getElementById('todoList');
list.innerHTML = '';
todos.forEach(todo => {
const li = document.createElement('li');
li.textContent = todo;
list.appendChild(li);
});
}
React:
function TodoList() {
const [todos, setTodos] = React.useState([]);
const [input, setInput] = React.useState('');
const addTodo = () => {
setTodos([...todos, input]);
setInput('');
};
return (
<div>
<input
value={input}
onChange={(e) => setInput(e.target.value)}
/>
<button onClick={addTodo}>Add</button>
<ul>
{todos.map((todo, index) => (
<li key={index}>{todo}</li>
))}
</ul>
</div>
);
}
Should You Learn React?
✅ Learn React if:
You build interactive web applications
You’re looking for a job (more jobs use React)
You need to maintain large applications
You work with a team
You want to build mobile apps later (React Native)
You enjoy component-based architecture
You want to join a thriving community
⚠️ Consider alternatives if:
You're building simple static websites
You're just starting with JavaScript
You need better SEO out of the box (Next.js might be better)
You prefer smaller bundle sizes (Svelte might be better)
You want full-stack features built-in (Angular might be better)
Getting Started
Go through the documentation IT’S REALLY GOOD.


Why struggle with file uploads? Pinata’s File API is your fix
Simplify your development workflow with Pinata’s File API. Add file uploads and retrieval to your app in minutes, without the need for complicated configurations. Pinata provides simple file management so you can focus on creating great features.
*A message from our sponsor.


Thank you to everyone who submitted 😃
RelyingEarth87, Classy-Cassy, mc-milo, ddat828, Micwir, ravener, pyGuy152, agentNinjaK, LaurelineP, SohamDandekar, dropbearII, JamesHarryT, Jk-frontEnd, SlothKai, tobiaoy, pavan-15-hub, codiling, and GiantMango.
Valid JavaScript Comments
In JavaScript, there are two types of comments:
Single-line comments start with
//
Multi-line or inline comments start with
/*
and end with*/
The input will be a sequence of //
, /*
and */
. Every /*
must have a */
that immediately follows it. To add, there can be no single-line comments in between multi-line comments in between the /*
and */
.
Create a function that returns True
if comments are properly formatted, and False
otherwise.
Examples
comments_correct("//////")
output = True
# 3 single-line comments: ["//", "//", "//"]
comments_correct("/**//**////**/")
output = True
# 3 multi-line comments + 1 single-line comment:
# ["/*", "*/", "/*", "*/", "//", "/*", "*/"]
comments_correct("///*/**/")
output = False
# The first /* is missing a */
comments_correct("/////")
output = False
# The 5th / is single, not a double //
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!

New video coming this week :)
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
Reply