- Sloth Bytes
- Posts
- 🦥What Are Pure Functions?
🦥What Are Pure Functions?
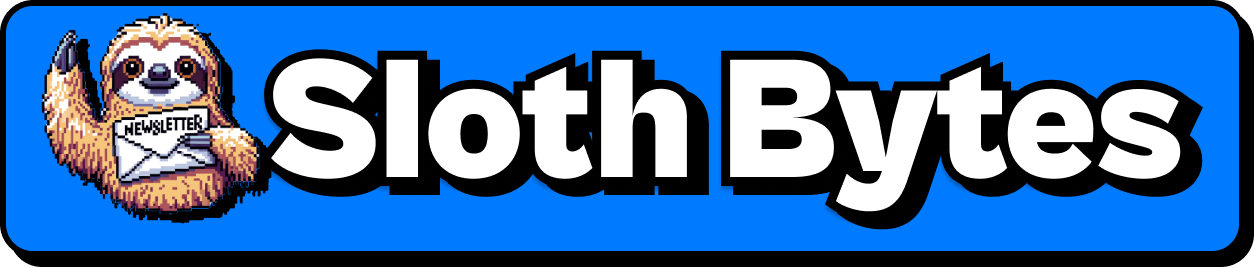
Hello friends!
Welcome to this week’s super late Sloth Bytes uh… I hope you had an amazing week.

Find out why 1M+ professionals read Superhuman AI daily.
AI won't take over the world. People who know how to use AI will.
Here's how to stay ahead with AI:
Sign up for Superhuman AI. The AI newsletter read by 1M+ pros.
Master AI tools, tutorials, and news in just 3 minutes a day.
Become 10X more productive using AI.

Pure Functions

So I’ve recently been studying a bit of functional programming because I wanted to see what was so good about it.
Functional bros really enjoy it (Haskell users…)
And one concept that really stood out to me is the idea of pure functions.
I’ve heard the term and a lot of people prefer writing functions like this and after reading about them, I definitely understand why.
It’s VERY simple and incredibly powerful.
It has a lot of benefits like reducing bugs, improving readability, and makes your code easier to test.
What Is a Pure Function?
Technical Explanation:
A pure function is a function that, given the same inputs, will always return the same output and it does not produce any observable side effects (like modifying global state, changing a file, or logging to the console).
Analogy:
It’s like a vending machine that always gives you the same snack for the same code. No matter how many times you press B2, you get chips. No surprises.
Example (JavaScript of course):
//Pure function: Same inputs, same outputs, no side effects.
function add(a, b) {
return a + b;
}
//Impure: Relies on external state and modifies it
let counter = 0;
function impureAdd(a) {
counter++;
return a + counter;
}
Why Use Pure Functions?
Predictability: No weird behavior. No guessing.
Testability: Super easy to unit test. Just pass inputs and assert outputs.
Debuggability: If something goes wrong, you know it’s the function, not some hidden state.
Reusability: Pure functions are modular and composable.
They’re also the building blocks of functional programming and are used heavily in languages like Haskell, Elm, and even modern React.
What the heck are Side Effects?
A side effect is anything your function does that isn’t returning a value:
Writing to a DB, mutating a variable, making an API call, updating the DOM, etc.
Side effects aren’t always bad! Sometimes they’re necessary.
But keeping your core logic pure and pushing side effects to the edge of your codebase is a clean, powerful design principle.
Bonus Tip: Combining Pure Functions
Once you start writing pure functions, you’ll find you can chain them together to do more complex work without losing clarity.
This is where you end up with fun syntax like this.
const double = x => x * 2;
const square = x => x * x;
const increment = x => x + 1;
const halve = x => x / 2;
const negate = x => -x;
const format = x => `Result: ${x}`;
const dramaticChain = [3, 5, 7, 9]
.map(double) // [6, 10, 14, 18]
.map(square) // [36, 100, 196, 324]
.map(increment) // [37, 101, 197, 325]
.map(halve) // [18.5, 50.5, 98.5, 162.5]
.map(negate) // [-18.5, -50.5, -98.5, -162.5]
.map(format); // ["Result: -18.5", ..., etc.]
console.log(dramaticChain);
Pretty fun right?
Might look annoying but if you break it down, it’s still small testable and predictable.
Pure functions won’t solve every problem, but they’re one of the simplest ways to write cleaner, more reliable code.
If you can make something pure, do it.
Because bugs love side effects and your future self will thank you.
Anyways, stay pure and keep coding.


Thanks for the feedback!



Thanks to everyone who submitted!
bakzkndd, Apoll011, papu163, AlePlaysDev, GabrielDornelas, juulCoding04, ShadowDara, Wakorithegreat, FredericoSRamos, JamesHarryT, numbersanalyst, epicawesomnes, porrrq, ariatheroyal, Heggol, Franspi-lol, RelyingEarth87, sanjayssrini, mmaarrius, and diegoteis1222.
Word Buckets
Write a function that divides a phrase into word buckets, with each bucket containing n
or fewer characters. Only include full words inside each bucket.
Examples
split_into_buckets("she sells sea shells by the sea", 10)
output = ["she sells", "sea shells", "by the sea"]
split_into_buckets("the mouse jumped over the cheese", 7)
output = ["the", "mouse", "jumped", "over", "the", "cheese"]
split_into_buckets("fairy dust coated the air", 20)
output = ["fairy dust coated", "the air"]
split_into_buckets("a b c d e", 2)
output = ["a", "b", "c", "d", "e"]
Notes
Spaces count as one character.
Trim beginning and end spaces for each word bucket (see final example).
If buckets are too small to hold a single word, return an empty list:
[]
The final goal isn't to return just the words with a length equal (or lower) to the given
n
, but to return the entire given phrase bucketized (if possible).
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!
If you want to be mentioned here, I’d prefer if you sent a GitHub link or Replit!

New Video is out!
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
What'd you think of today's email? |
Want to advertise in Sloth Bytes?
If your company is interested in reaching an audience of developers and programming enthusiasts, you may want to advertise with us here.
Reply