- Sloth Bytes
- Posts
- 𦄠OOP Pillar #4: Polymorphism For Dummies
𦄠OOP Pillar #4: Polymorphism For Dummies
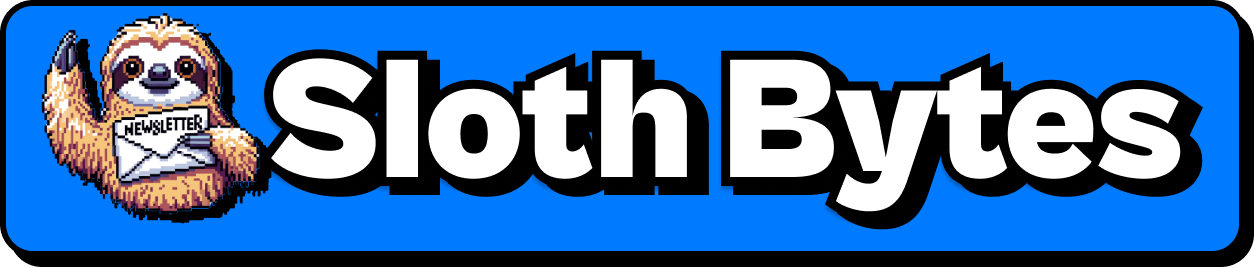
Hello friends!
Welcome to this weekās Sloth Bytes.
I hope you had a good week š

Sloths are nocturnal
Two-toed sloths are completely nocturnal. Although three-toed sloths are both diurnal and nocturnal, theyāre largely inactive during the day.

OOP Pillar #4: Polymorphism

What is Polymorphism?
Polymorphism describes a pattern in object oriented programming in which classes have different functionality while sharing a common interface. (I stole it from here)
Why Polymorphism Matters
Increases code flexibility and reusability
Simplifies code structure
Enables more intuitive design of complex systems
Facilitates easier maintenance and scalability
Key Concepts:
Method Overriding: Redefining a method in a subclass
Method Overloading: Multiple methods with the same name but different parameters
Interface Implementation: Different classes implementing the same interface
Dynamic Method Dispatch: Runtime decision of which method to call
We can use the same example as last weekās post to simplify our code a bit.
Polymorphism can be complex but weāll keep it simple.
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def speak(self):
return f"{self.name} is an animal."
class Dog(Animal):
#Method overriding - we're redefining the method
def speak(self):
return f"{self.name} is a dog."
#inherited the self.name from the Animal class
class Cat(Animal):
#Method overriding - we're redefining the method
def speak(self):
return f"{self.name} is a cat."
#inherited the self.name from the Animal class
# Usage
animal = Animal("goofy", 5)
dog = Dog("Buddy", 10)
cat = Cat("Whiskers", 5)
print(animal.speak()) # Output: Animal is an animal.
print(dog.speak()) # Output: Buddy is a dog.
print(cat.speak()) # Output: Whiskers is an cat.
class Animal {
String name;
int age;
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public String speak() {
return name + " is an animal.";
}
}
class Dog extends Animal {
public Dog(String name, int age) {
super(name, age); //Allows us to access parent class info.
}
// Method overriding - we're redefining the method
public String speak() {
return name + " is a dog.";
}
}
// Subclass/Child class
class Cat extends Animal {
public Cat(String name, int age) {
super(name, age); //Allows us to access parent class info.
}
// Method overriding - we're redefining the method
public String speak() {
return name + " is a cat.";
}
}
// Usage
public class Main {
public static void main(String[] args) {
// Usage
Animal animal = new Animal("goofy", 5)
Dog dog = new Dog("Buddy", 10);
Cat cat = new Cat("Whiskers", 5);
System.out.println(animal.speak());
// Output: goofy is an animal.
System.out.println(cat.speak());
// Output: Whiskers is a cat.
System.out.println(dog.speak());
// Output: Buddy is an animal.
System.out.println(cat.speak());
// Output: Whiskers is an animal.
}
}
#include <iostream>
#include <string>
using namespace std;
// Base class
class Animal {
public:
string name;
int age;
Animal(string name, int age) : name(name), age(age) {}
void speak() {
cout << name << " is an animal.\n";
}
};
// Derived class
class Dog : public Animal {
public:
Dog(string name, int age) : Animal(name, age) {}
void speak() {
cout << name << " is a dog.\n";
}
};
// Derived class
class Cat : public Animal {
public:
Cat(string name, int age) : Animal(name, age) {}
void speak() {
cout << name << " is a cat.\n";
}
};
int main() {
Animal animal("Goofy", 5);
Dog dog("Buddy", 10);
Cat cat("Whiskers", 5);
animal.speak(); // Output: Goofy is an animal
dog.speak(); // Output: Buddy is a dog
cat.speak(); // Output: Whiskers is a cat
return 0;
}
Even though dog and cat are derived from the animal class, their speak methods behave different.
Thereās also different types of polymorphism and hereās an easy way to tell which one is happening:
Compile time polymorphism - method overloading
Runtime polymorphism - method overriding
Best Practices:
Design clear and concise interfaces
Use method overriding carefully.
Leverage polymorphism for flexible code design
Combine with other OOP principles for robust systems
Common Pitfalls:
Overuse leading to unnecessarily complex hierarchies
Confusion between overloading and overriding
Performance overhead in some languages
Real-World Analogy: Think of a universal remote control. It can operate various devices (TV, DVD player, sound system) through a common interface, despite each device having different underlying operations.
Remember: Polymorphism is about providing a single interface to entities of different types. It's a powerful tool for creating flexible and scalable code.
Quick Tip: When designing systems, think about what behaviors different objects might share. These common behaviors are excellent candidates for polymorphic interfaces.




Thank you to everyone who submitted last week š
coasterkolja, FragileBranch, Drodriguezponce1, ddat828, ravener, sloprope (sent 2 solutions I respect that), VSparl, sarolta-nemeth, RelyingEarth87, Neko0kami, DevonKirby, and the last 2:


Nearest Vowel
Given a letter, created a function which returns the nearest vowel to the letter. If two vowels are equal distance to the given letter, return the earlier vowel.
Examples
nearest_vowel("b")
output = "a" # closest vowel is a
nearest_vowel("s")
output = "u" # closest vowel is u
nearest_vowel("c")
output = "a" # closest vowel is a
nearest_vowel("i")
output = "i" # i is a vowel, so return itself
Notes
All letters will be given in lowercase.
There will be no alphabet wrapping involved, meaning the closest vowel to "z" should return "u", not "a".
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if youāre on the web version leave a comment!

Consistent uploads???
Check out the new video I posted if you havenāt already š
Another video is being cooked up
Yep Iām working on the next video hoping to post it this week/early next week.
Thatās all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply