- Sloth Bytes
- Posts
- 🦥 OOP Pillar #3: Inheritance For Dummies
🦥 OOP Pillar #3: Inheritance For Dummies
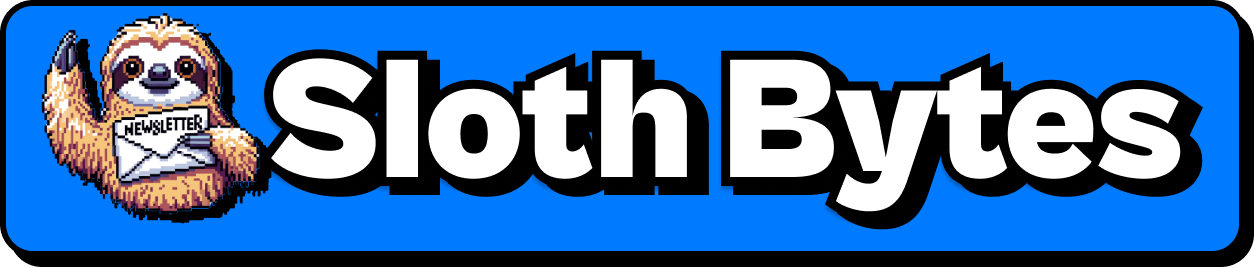
Hello friends!
Welcome to this week’s Sloth Bytes.
Hope you had a great week!

Sloths spend up to 90% of their lives upside down
Studies show this is possible because their organs are attached to their rib cage, so they don’t weigh down on their lungs.
Unlike us, a sloth can hang upside down without affecting their breathing.

OOP Pillar #3: Inheritance

What is Inheritance?
Inheritance is when a new class takes on or you know… INHERITS the properties and methods of an existing class. This helps in reusing code and building a structure where classes are related to each other.
Why Inheritance Matters
Code reusability
Establishes a clear hierarchy
Allows polymorphism (I’ll talk about that next week 😁)
Reduces redundant code
Key Concepts:
Superclass (Parent class): The class being inherited from
Subclass (Child class): The class that inherits
Override: Redefining a method in a subclass
Extend: Adding new methods or properties in a subclass
Python Example:
class Animal:
def __init__(self, name, age):
self.name = name
self.age = age
def speak(self):
return f"{self.name} is an animal."
class Dog(Animal):
def speak_dog(self):
return f"{self.name} is a dog."
#inherited the self.name from the Animal class
class Cat(Animal):
def speak_cat(self):
return f"{self.name} is a cat."
#inherited the self.name from the Animal class
# Usage
dog = Dog("Buddy", 10)
cat = Cat("Whiskers", 5)
print(dog.speak_dog()) # Output: Buddy is a dog.
print(cat.speak_cat()) # Output: Whiskers is a cat.
print(dog.speak()) # Output: Buddy is an animal.
print(cat.speak()) # Output: Whiskers is an animal.
Java Example:
// superclass/Parent class
class Animal {
String name;
int age;
public Animal(String name, int age) {
this.name = name;
this.age = age;
}
public String speak() {
return name + " is an animal.";
}
}
// Subclass/Child class
class Dog extends Animal {
public Dog(String name, int age) {
super(name, age); //Allows us to access parent class info.
}
// Method specific to Dog
public String speakDog() {
return name + " is a dog.";
}
}
// Subclass/Child class
class Cat extends Animal {
public Cat(String name, int age) {
super(name, age); //Allows us to access parent class info.
}
public String speakCat() {
return name + " is a cat.";
}
}
// Usage
public class Main {
public static void main(String[] args) {
// Usage
Dog dog = new Dog("Buddy", 10);
Cat cat = new Cat("Whiskers", 5);
System.out.println(dog.speakDog());
// Output: Buddy is a dog.
System.out.println(cat.speakCat());
// Output: Whiskers is a cat.
System.out.println(dog.speak());
// Output: Buddy is an animal.
System.out.println(cat.speak());
// Output: Whiskers is an animal.
}
}
Benefits of Inheritance:
Code reusability
Logical organization of code
Runtime polymorphism
Easier maintenance and updates
Best Practices:
Use inheritance when one thing "is a" type of another thing. ("is-a" relationships)
Keep inheritance hierarchies shallow.
Override methods carefully
Use composition instead of inheritance when it makes sense.
Composition = “has-a”.
Inheritance = “is-a”.
ex: A building IS A house, but a bathroom is NOT a building or a house. However, a house HAS A bathroom.
Common Pitfalls:
Overuse leading to complex hierarchies
Tight coupling between classes
Breaking encapsulation in subclasses
Real-World Analogy: Think of a family tree. Children inherit traits from their parents, but may also have unique characteristics.
Remember: Inheritance is powerful, but use it wisely. Not every relationship between classes needs to be an inheritance relationship.
Quick Tip: When deciding whether to use inheritance, ask: "Does this new class need everything from the parent class, or just some things?" If it's the latter, consider composition instead.




Thank you to everyone who submitted last week!
Love to see more people submitting.
ChicIceCream, ddat828, FragileBranch, kwame-Owusu, afiqzudinhadi, DevonKirby, Dupamin, tobiaoy, sloprope, ravener, RelyingEarth87, JSivic25, Neko0kami, VSparl, sarolta-nemeth, and last one:

Let’s do some practice with 2d arrays 😁
Where’s Waldo
Return the coordinates ([row, col]
) of the element that differs from the rest.
Examples
whereIsWaldo([
["A", "A", "A"],
["A", "A", "A"],
["A", "B", "A"]
])
output = [3, 2] #B is different from the rest.
whereIsWaldo([
["c", "c", "c", "c"],
["c", "c", "c", "d"]
])
output = [2, 4] # D is different from the rest.
whereIsWaldo([
["O", "O", "O", "O"],
["O", "O", "O", "O"],
["O", "O", "O", "O"],
["O", "O", "O", "O"],
["P", "O", "O", "O"],
["O", "O", "O", "O"]
])
output = [5, 1] #P is different from the rest.
Notes
The given array will always be a square or rectangle.
Rows and columns are 1-indexed (not zero-indexed).
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!

Video finally got posted
If you haven’t already check out the new video 😄
Working on the next video!
Next video is how to use AI to program better/faster and how to use it to learn faster. So stay tuned!
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply