- Sloth Bytes
- Posts
- š¦„OOP Pillar #2: Encapsulation For Dummies
š¦„OOP Pillar #2: Encapsulation For Dummies
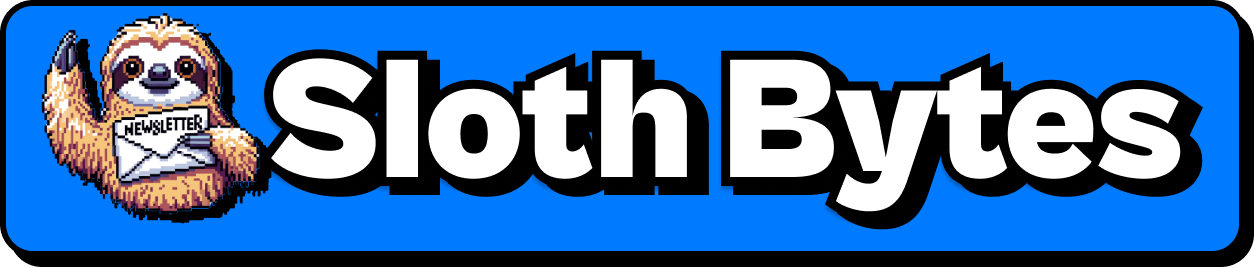
Hello friends!
Welcome to this weekās Sloth Bytes. I hope you had a great week.

Sloths digest really slow
Sloths have a four-part stomach that very slowly digests the tough leaves they eat, it can sometimes take up to a month for them to digest a meal. Digesting this slow means they have low energy which is one of the reasons why theyāre so slow.

OOP Pillar #2: Encapsulation

What is Encapsulation?
Encapsulation means putting data and the methods that work on that data together in one place. It also means hiding how the object works from the outside.
Why Encapsulation Matters
Protects data from unauthorized access
Reduces system complexity
Increases code flexibility and maintainability
Promotes data integrity
Key Concepts:
Data Hiding:
Use private variables to restrict direct access
Provide public methods (getters/setters) to interact with the data
Access Modifiers:
public: Accessible from anywhere
private: Accessible only within the class
protected: Accessible within the class and its subclasses
Python Example:
class BankAccount:
def __init__(self):
self.__balance = 0 # Private variable
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return True
return False
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
return True
return False
def get_balance(self):
return self.__balance
# Usage
account = BankAccount()
account.deposit(100)
print(account.get_balance()) # Output: 100
print(account.__balance) # AttributeError: 'BankAccount' object has no attribute '__balance'
Java Example:
public class BankAccount {
private double balance;
public boolean deposit(double amount) {
if (amount > 0) {
this.balance += amount;
return true;
}
return false;
}
public boolean withdraw(double amount) {
if (amount > 0 && amount <= this.balance) {
this.balance -= amount;
return true;
}
return false;
}
public double getBalance() {
return this.balance;
}
}
// Usage
BankAccount account = new BankAccount();
account.deposit(100);
System.out.println(account.getBalance()); // Output: 100.0
// System.out.println(account.balance); // Compilation error: balance has private access
Benefits of Encapsulation:
Protects the integrity of data
Allows for code refactoring without affecting other parts
Provides a clear interface for object interaction
Enhances security by controlling access to data
Best Practices:
Use private variables for internal state
Provide public methods for necessary interactions
Implement validation in setter methods
Use meaningful names for methods
Common Pitfalls:
Over-encapsulation: Making everything private
Under-encapsulation: Exposing too much internal data
Ignoring encapsulation principles in small projects
Real-World Analogy
Think of a vending machine. You can't directly access the items or money inside. You interact with it through a specific interface (buttons, coin slot) that controls access to its internal components.
Remember: Encapsulation is about controlling access to the internal details of an object. It's not just about making variables private, but about providing a well-defined interface for interacting with an object.
Quick Tip: When designing a class, ask yourself: "What does this object need to expose to function properly?" Everything else can potentially be encapsulated.
Encapsulation is a powerful tool for creating robust and maintainable code. By mastering this concept, you'll be able to design more secure and flexible systems.




Thank you to everyone who submitted last week!
A lot of people submitted againš
ChicIceCream, ddat828, sloprope, Eriq606, Yoshlix, RelyingEarth87, hotshotberad, VSparl, sarolta-nemeth, Katzentante, kwame-Owusu, afiqzudinhadi, Shraddha Patel, ravener, and last one:

Count the Smiley Faces :)
Create a function that takes an array of strings and return the number of smiley faces contained within it. These are the components that make up a valid smiley:
A smiley has eyes. Eyes can be : or ;
A smiley has a nose but it doesn't have to. A nose can be - or ~
A smiley has a mouth which can be ) or D
No other characters are allowed except for those mentioned above.
Examples
countSmileys([":)", ";(", ";}", ":-D"])
output = 2 # the two smileys are ":)" and ":-D"
countSmileys([";D", ":-(", ":-)", ";~)"])
output = 3 #The three smileys are ";D", ":-)", and ";~)"
countSmileys([";]", ":[", ";*", ":$", ";-D"])
output = 1 #The smiley is ";-D"
Notes
You will always be given an array as input.
An empty array should return 0.
The order of each facial element will always be the same.
Noses are optional (e.g.
:)
and:-)
are both valid).
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if youāre on the web version leave a comment!

Video is done š
The video is finally done, but unfortunately I need to get it approved. HOPEFULLY ITS FINALLY HERE THIS WEEK.
Iām also working on the next video, so it shouldnāt take a month lol.
Thatās all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply