- Sloth Bytes
- Posts
- 🦥 OOP Pillar #1: Abstraction For Dummies
🦥 OOP Pillar #1: Abstraction For Dummies
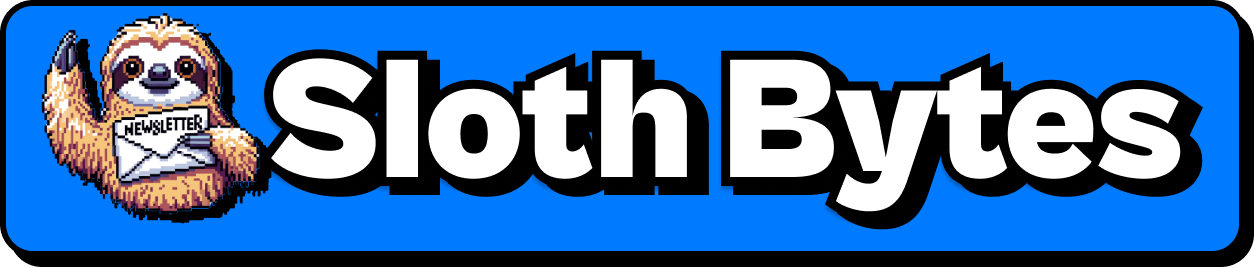
Hello friends!
Welcome to this week's Goofy Bytes.
Hope you all had a great week 😄

Sloths are basically vegetarians.
Sloths are herbivores. This means they eat lots of leaves and fruit, but no meat. So, you’ll never have to worry about a sloth trying to eat you.

OOP Pillar #1: Abstraction

What is Abstraction?
Abstraction is the process of hiding complex implementation details and showing only the essential features of an object. It's about creating a simplified model of a complex system.
Why Abstraction Matters:
Reduces complexity
Improves code maintainability
Allows focus on high-level functionality
Promotes code reusability
Key Concepts:
Abstract Classes:
Cannot be instantiated
May contain abstract methods (methods without a body)
Serve as a blueprint for other classes
Interfaces:
Define a contract for classes to implement
Contain only abstract methods
Allow for multiple inheritance in languages like Java
Python Example:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return "Woof!"
class Cat(Animal):
def make_sound(self):
return "Meow!"
# Usage
dog = Dog()
print(dog.make_sound()) # Output: Woof!
Real-World Analogy: Think of a car. You don't need to know how the engine works to drive it. The complexity is abstracted away, leaving you with simple controls (steering wheel, pedals, etc.).
Another terrible example
Imagine you're building a web application that requires user authentication. The process involves a lot of complex operations:
User registration
Login
Password hashing
JWT (JSON Web Token) generation and validation
Database interactions
Session management
Logout
Without Abstraction (building from scratch like a chad):
def register_user(username, password):
# Complex logic for password hashing
hashed_password = complex_hash_function(password)
# Database interaction to store user
database.store_user(username, hashed_password)
# Generate JWT
token = generate_jwt(username)
# Set cookies
set_cookie(token)
def login_user(username, password):
# Retrieve user from database
stored_user = database.get_user(username)
# Complex logic for password verification
if verify_password(password, stored_user.hashed_password):
# Generate JWT
token = generate_jwt(username)
# Set cookies
set_cookie(token)
else:
raise AuthenticationError("Invalid credentials")
# ... more complex functions for logout, password reset, etc.
That's a lot of code to review and a lot of it is already abstracted.
If I have my unpaid intern with no coding experience try to implement authentication it would be difficult.
You can use abstraction to simplify the process.
A common example is that nowadays everyone uses some authentication library to handle this for them.
from some_random_auth_library import AuthManager
fake_auth_manager = AuthManager()
def register_user(username, password):
fake_auth_manager.register(username, password)
def login_user(username, password):
fake_auth_manager.login(username, password)
def logout_user():
fake_auth_manager.logout()
We hid the implementation details in the AuthManager class, so now it’s much easier to look at and implement. The unpaid intern just needs to add one line of code for each method, so there’s no way they could mess it up (hopefully).
The intern only needs to know that this will handle registration, login, and logout. They don’t need to worry about how it works.
Sure they can view the class if they want, but it’s not necessary.
Benefits of Abstraction:
Simplifies complex systems
Hides unnecessary details
Provides a clear and easy-to-use interface
Allows for code evolution without affecting other parts
Best Practices:
Keep abstractions at an appropriate level
Use meaningful names for abstract classes and methods
Don't over-abstract. Balance abstraction with practicality
Common Pitfalls:
Creating abstractions too early
Making things too complicated
Confusing abstraction with encapsulation
Remember: Abstraction is about creating a simplified model of reality. It's not about making things vague, but about making them clear and manageable.
Quick Tip: When designing a system, start by identifying the essential characteristics and behaviors. These form the basis of your abstractions.
Abstraction is the foundation that builds robust and flexible OOP systems. By mastering this concept, you'll be well on your way to writing cleaner, more maintainable code.

Cut Through Noise with The Flyover!
The Flyover offers a refreshing alternative to traditional news. Our talented editors meticulously curate the day's top stories, keeping you informed and ready to win your day. Join over 600,000 readers who trust The Flyover's unbiased insights!




Thank you to everyone who submitted last week! It was a lot…
Shraddha Patel, meral-z, sloprope, sarolta-nemeth, Eriq606, unkokaeru, Yoshlix, JSivic25, RelyingEarth87, ravener, ddat888, rushi-001, kwame-Owusu, Drodriguezponce1, Codin-bee, and so much more…
Sort by the Letters
Write a function that sorts each string in a list by the letter in alphabetic ascending order (a-z).
Examples
sort_by_letter(["932c", "832u32", "2344b"])
output = ["2344b", "932c", "832u32"]
sort_by_letter(["99a", "78b", "c2345", "11d"])
output = ["99a", "78b", "c2345", "11d"]
sort_by_letter(["572z", "5y5", "304q2"])
output = ["304q2", "5y5", "572z"]
sort_by_letter([])
output = []
#here's your starting point :)
def sort_by_letter(arr):
Notes
Each string will only have one (lowercase) letter.
If given an empty list, return an empty list.
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!

So uhhh new video uhhhhhh
Yeah this video is SO CLOSE TO BEING DONE. I’m hoping I can upload it this week.
Here’s a sneak peek:

lol you’re welcome.
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply