- Sloth Bytes
- Posts
- 🦥 Understanding Object-Oriented Programming (OOP)
🦥 Understanding Object-Oriented Programming (OOP)
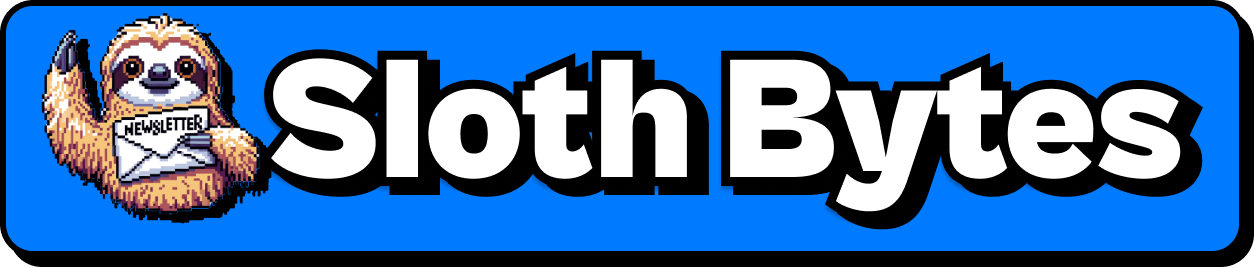
Hello friends!
Welcome to this week's Sloth Bytes.
There won’t be a book of the week since I’ve been slacking…
Whoops.
I’m also thinking of removing weekly challenges/projects altogether to focus more on information. what do you think?
Remove Weekly Challenge/Project?Should I remove it to focus more on programming information? |
Once again don't hesitate to hit reply, I’m always open to suggestions!

Sloths can fall 100 feet without injury
Sloths are anatomically designed to fall out of trees.
On average, a sloth will fall out of a tree once a week for its entire life. But don’t worry, all sloths are anatomically designed to fall and survive.

Object-Oriented Programming (OOP)

What is this?
Object-oriented programming (OOP) is a way of organizing your code by using 'objects' and “classes” to represent data and methods. This makes it easier to manage and scale your code.
What the heck are objects and classes
Classes: The blueprints that define the structure and capabilities of objects.
Objects: The creations from these blueprints.
The difference between objects and classes
For example, we create a Car
class with these attributes:
Color
Size
Number of wheels
This class acts as the blueprint.
Using this blueprint, you can create an actual car, which is the object.
In programming, this object is referred to as an "instance" of the Car
class.
This means whenever you create an object from a class, you’re creating an “instance” of the class.
Main Components of a Class (THIS IS IMPORTANT)
Let’s get a bit more technical. I’ll use code examples to help you.
I’ll do this in Java since everything’s a class and object.
Instance Variables
This contains the objects information.
There’s a lot of names for it: attributes, properties, fields, etc.
Pick whichever feels right to you.
Simple Terms: Variables inside a class.
public class Car {
// Instance variables
public String color;
public String size;
public int numberOfWheels;
}
Methods
Methods are the actions that the object can do.
Simple terms: Functions inside a class.
public class Car {
// "Method" for driving
public void drive() {
System.out.println("The car is driving.");
}
// "Method" for braking
public void brake() {
System.out.println("The car has stopped.");
}
}
Constructor
The constructor creates or “initializes” the object.
It’s the starting point.
public class Car {
// Instance variables
public String color;
public String size;
public int numberOfWheels;
// Constructor to "initialize" the Car object
public Car() {
this.color = "red";
this.size = "large like your mom";
this.numberOfWheels = "20";
}
}
Complete Example
public class Car {
// Instance variables
public String color;
public String size;
public int numberOfWheels;
// Constructor to "initialize" the Car object
public Car(String color, String size, int numberOfWheels) {
this.color = color;
this.size = size;
this.numberOfWheels = numberOfWheels;
}
// "Method" for driving
public void drive() {
System.out.println("The " + color + " car is driving.");
}
// "Method" for braking
public void brake() {
System.out.println("The " + color + " car has stopped.");
}
}
// An example of creating an "instance" of Car
Car myCar = new Car("Red", "Compact", 4);
myCar.drive();
myCar.brake();
Why is this important
Easier to Manage: OOP lets you manage your code in a clean, modular way.
Saves Time: Once you create an object, you can reuse it in other parts of your program without rewriting code.
Secure: It helps keep data safe and prevents it from being accidentally changed by other parts of your program.
Flexible: As your program grows, OOP makes it easier to make changes and add new features.


What is this?
Scribe is a tool that’ll turn any process into a step-by-step guide, instantly.
It’s a very helpful tool if you need to show somebody how to do something.
Benefits
✅ Save time: Document your processes 15x faster. No more writing steps or uploading screenshots.
✅ Spread knowledge: Find answers to your questions 67% faster.
✅ Increase success: Boost your team’s productivity by 25%.


Calculate Damage - Beginner
Create a function that takes damage
and speed
(attacks per second) and returns the amount of damage after a given time
.
Examples
damage(40, 5, "second") ➞ 200
damage(100, 1, "minute") ➞ 6000
damage(2, 100, "hour") ➞ 720000
Return "invalid" if damage
or speed
is negative.
How To Submit Answers
Reply with this:
A link to your solution (github, personal blog, portfolio, etc)
A link to your post on Twitter, Linkedin, or any social platform you use.

New Video is almost done I swear…
Here’s a sneak peek 🙂

This is basically what I’ve been working on this whole week which means uhhh…
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply