- Sloth Bytes
- Posts
- 🦥 Debugging Techniques You Should Know
🦥 Debugging Techniques You Should Know
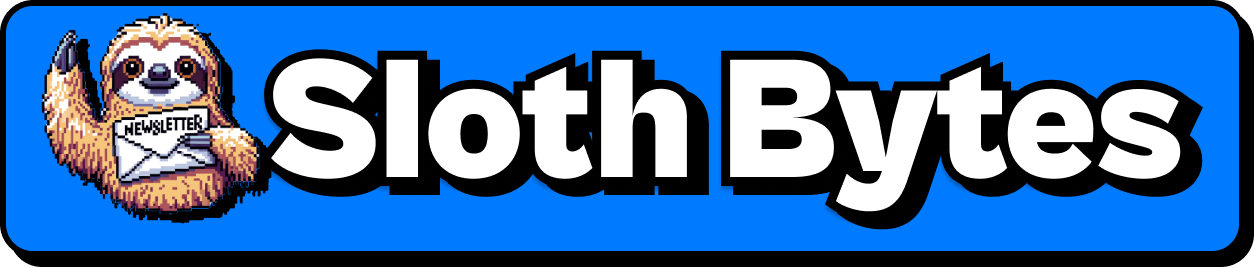
Hello friends!
Welcome to this week’s Sloth Bytes. Hope you had an amazing week!

Sloths sleep as long as humans
People think that sloths sleep for 20 hours a day, but that’s a myth! Wild sloths only sleep for about 8 to 10 hours each day, about the same as humans.

Debugging Techniques

What is debugging?
Debugging is the process of finding and fixing errors (bugs) in your code. It's an essential skill for every programmer because nobody can write perfect code.
Why is debugging important?
Quickly identify and fix issues
Improves code quality
Understand your code better
Learn from mistakes and prevent future bugs
Techniques
Print Statements: A classic technique every programmer does. Add temporary print statements to display variable values and track execution flow.
How it helps: This helps you see what's happening inside your code as it runs.
When to use: Use it for quick checks or when you're feeling lazy (like me).
Example:
def calculate_sum(a, b):
# Debug print
print(f"a: {a}, b: {b}")
# Debug print
result = a + b
print(f"result: {result}")
return result
sum = calculate_sum(5, 3)
print(f"Sum: {sum}")
Using a Debugger: A tool that allows you to pause code execution, step through it line by line, and inspect variable values.
How it helps: Gives detailed insight into code execution and variable states at any point.
When to use: For complex bugs or when you need to closely examine code behavior.
Custom Error Messages: Create custom error messages using your programming language’s error handling methods.
How it helps: Provides more specific error information and allows handling of expected error conditions.
When to use: When you want to provide more context about errors in your code. If you do web development, this is usually how you send error messages to the front end.
Example:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero you idiot")
return a / b
try:
result = divide(10, 0)
except ValueError as e:
print(f"Error: {e}")
#Result: output Traceback (most recent call last): File "example.py", line 5, in <module> result = 10 / 0 ZeroDivisionError: division by zero you idiot
Assertions: Use assertions to check for conditions that should never occur in your code.
How it helps: Catches logical errors early in the development process.
When to use: During development and testing to verify assumptions about your code.
Example:
def calculate_average(numbers):
#This part right here
assert len(numbers) > 0, "List cannot be empty bozo"
return sum(numbers) / len(numbers)
calculate_average([])
#Result: AssertionError: List cannot be empty bozo
Rubber Duck Debugging: Explain your code line by line to somebody, or if you don't have friends, to some object (like a rubber duck).
How it helps: Forces you to articulate your logic, often revealing flaws in your thinking.
When to use: When you're stuck and need a fresh perspective on your code.
Logging: Use logging libraries to record information about your program's execution.
How it helps: Provides a persistent record of program behavior, useful for tracking issues over time.
When to use: In larger applications, when debugging issues that occur randomly, or if you need a history of logs.
Example:
import logging
logging.basicConfig(level=logging.DEBUG)
def divide(a, b):
logging.debug(f"Dividing {a} by {b}")
if b == 0:
logging.error("Division by zero!")
return None
return a / b
result = divide(10, 2)
logging.info(f"Result: {result}")
#Result:
# DEBUG:root:Dividing 10 by 2
# INFO:root:Result: 5.0
My Debugging Process
Reproduce the bug: Find a way trigger the error
Locate the error: Identify where the problem occurs
Analyze the problem: Understand why it's happening
Fix the bug: Make necessary code changes
Test the fix: Ensure the bug is resolved and no new issues are introduced
Update your code: Commit the changes and document if necessary
Tips for Effective Debugging
Stay calm and patient: Debugging can be frustrating, but a clear mind helps
Use version control: Track changes and revert if needed
Comment your code: Makes it easier to understand your logic later
Take breaks: A fresh perspective can reveal overlooked issues
Ask for help: Don't hesitate to seek advice from colleagues or online communities.
Remember, debugging is a skill that improves with practice. Each bug you solve makes you a better programmer!

Binary Search

What is Binary Search?
Binary search is an efficient algorithm for finding a target value in a sorted array. It works by repeatedly dividing the search interval in half.
Why is it important?
Extremely efficient for large datasets
Common interview topic
How it works
Start with the middle element of the sorted array
If the target value is equal to the middle element, we're done
If the target is less than the middle element, repeat the search on the left half
If the target is greater than the middle element, repeat the search on the right half
Repeat until the target is found or it's clear the target isn't in the array
Time Complexity
O(log n) - much faster than linear search O(n) for large datasets
Basic Implementation (Python):
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
target = 7
result = binary_search(sorted_array, target)
print(f"Target {target} found at index: {result}")
Key Points to Remember
The array must be sorted for binary search to work
It's much faster than linear search for large datasets
Can be implemented iteratively (as above) or recursively
Be careful of integer overflow when calculating mid point (use
left + (right - left) // 2
for very large arrays)
Tips for Interviews
Always ask if the array is sorted
Consider edge cases (empty array, target not in array)
Think about how to handle duplicates
Be prepared to explain the time and space complexity




Thank you to everyone who submitted last week!
Vijaychandra2, Anand Prabhu, 220241, CarolinaFalcao, QueenlyHeart, ddat828, IshanKumar22, Codin-bee, Nuc1earOwl, Shraddha Patel, ravener, Addleo, RelyingEarth87, and many more (You all are awesome. Sorry if you were left out; there are a lot of submissions.)
Binary Search Practice
Given a sorted array of integers and a target integer, find the first occurrence of the target and return its index.
Return -1 if the target is not in the array.
Examples
#Input:
arr = [1, 3, 3, 3, 3, 6, 10, 10, 10, 100]
target = 3
find_first_occurrence(arr,target) # Return 1
#Explanation: The first occurrence of 3 is at index 1.
#Input:
arr = [2, 3, 5, 7, 11, 13, 17, 19]
target = 6
find_first_occurrence(arr,target) # Return -1
#Explanation: 6 does not exist in the array.
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)

Published that yap session
Yes, I posted the video I teased last week. If you haven't seen it yet, check it out.
Back to working on the AI project
Spent most of last week editing, so now I’m back to working on this project. I keep getting ideas for it, but I’m doing my best to stay on track (I won’t let feature creep win).
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
See you all next week.
Reply