- Sloth Bytes
- Posts
- 🦥 CORS: The Most Annoying Web Problem
🦥 CORS: The Most Annoying Web Problem
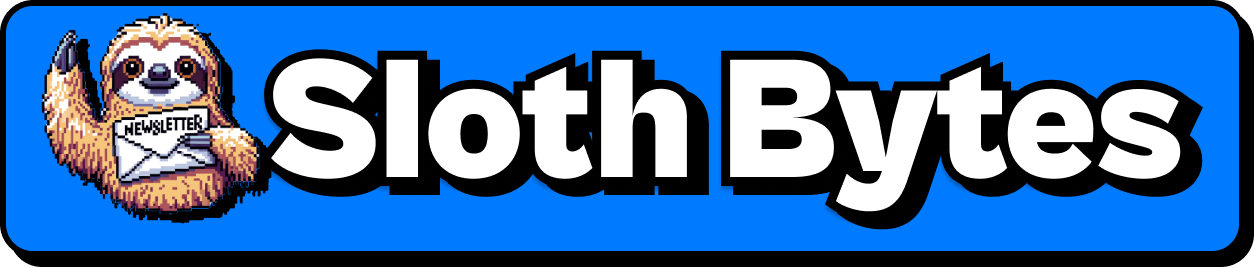
Hello friends!
Welcome to this week’s Sloth Bytes. I hope you had a weird week 😉
Did you do me that favor?
If you already filled it out last week, you can uh… skip this and enjoy the today’s newsletter.
If you didn’t read last weeks Sloth bytes or skipped my welcome email… (why?)
I recently made a welcome survey for the newsletter.
The survey helps me improve the newsletter by knowing what programming languages you use, what areas you’re interested in, and how you learn.
It’s a very quick survey, it’ll only take 30 seconds.
It's just 8 quick questions about your programming experience, interests, and preferred learning formats. No essay questions, I promise!
This will be the last week I mention it (Unless a lot of you skip it…)

There’s a reason 400,000 professionals read this daily.
Join The AI Report, trusted by 400,000+ professionals at Google, Microsoft, and OpenAI. Get daily insights, tools, and strategies to master practical AI skills that drive results.

CORS: The Most Annoying Web Problem

I remember when I first started learning full-stack development.
I was so excited to finally build both frontend and backend.
After tweaking my poorly made backend code, I finally had my API ready to test. Feeling confident, I fired up my frontend, made the call to amazing 10x developer API I crafted, and then BAM:

And that my friends… was my first encounter with CORS.
🧐 First things first: What exactly is CORS?
Cross-origin resource sharing (CORS) is a standardized mechanism allowing web apps to request resources from different domains safely.
Think of it like an exclusive VIP list: your browser uses this list to decide if a website is allowed access to external resources.
The browser has a built-in feature called same-origin policy where a website will only accept requests from the “same origin” which means:
Same domain
Same protocol
Same port
If that website domain doesn’t follow ANY of those 3 rules, then it’s a “different origin” and the browser will block those requests by default to prevent malicious attacks, but this can also stop legitimate, harmless uses (like trying to test my terrible backend code).
Quick same-origin policy example:
If your app runs at:
http://example.com:80
Here’s what happens if you try to make a request to these domains with same-origin policy active:
✅
http://example.com:80/page.html
(same origin)❌
https://example.com
(different protocol)❌
http://example.com:3000
(different port)❌
http://otherdomain.com
(different domain)
Yeah… it’s pretty picky (for good reason!)
🚧 Why does CORS exist?
Before CORS, malicious sites could execute Cross-Site Request Forgery (CSRF) attacks, tricking your browser into performing unwanted actions on your behalf.
For example, a malicious website could trick your browser into transferring money or leaking sensitive data.
To prevent this, browsers now strictly enforce the same-origin policy.
⚙️ How does CORS work?
I’ll be taking this example straight from AWS article on CORS
Let’s say we have a website called: news.example.com.
This site wants to access resources from an API at partner-api.com.
Developers at partner-api.com need to configure CORS headers on their server by adding:
Access-Control-Allow-Origin: https://news.example.com
This sets up CORS so that news.example.com can ask for resources from partner-api.com.
Each time a request is made, partner-api.com will reply with
Access-Control-Allow-Credentials : "true"
This tells the browser that communication between both domains allowed and permits cross-origin access.
If you want grant access to multiple origins, use a comma-separated list or use the wildcard character *. This grants access to everyone.
Access-Control-Allow-Origin: *
🚩 Preflight requests for complex HTTP calls
Some requests trigger an extra security check called a preflight request.
This happens if:
You’re using methods like PUT, PATCH, DELETE
Custom headers or certain content-types are involved.
How To Handle CORS
CORS is pretty easy to handle with modern day programming languages/tools.
They usually have a built in/community maintained package that lets you set it up easily:
JavaScript (Express + Node)
const express = require('express');
//You can install this with npm install cors
const cors = require('cors');
const app = express();
app.use(cors({
origin: 'http://localhost:3000', // specific origin or '*' for all
methods: 'GET,POST,PUT,DELETE',
credentials: true
}));
app.listen(3001, () => console.log('Server running on port 3001'));
Python (Flask)
from flask import Flask
//You can install this with pip install flask-cors
from flask_cors import CORS
app = Flask(__name__)
CORS(app, resources={r"/api/*": {"origins": "http://localhost:3000"}})
@app.route('/api/data')
def data():
return {"message": "Hello, CORS"}
app.run(port=5000)
Fun fact: If you switched to web sockets, CORS won’t happen… (yeah think about that)
Next time CORS tries to ruin your day, just smile and handle it like the coding champ you are.
If you want to learn more about CORS, then check out the mdn docs here.

Sloths have the slowest metabolic rate of any non-hibernating land mammal.



Thank you to everyone who submitted! A LOT of you submitted this week 😁
ShadowDara, papu163, RelyingEarth87, JamesHarryT, ravener, ghostgamr, Repr-dev, Raufirzaman, Franspi-lol, Pennicillina, Dhwani1401, Dhwani1401, josericardo-fo, FredericoSRamos, aviral-trivedi, JustVal-su, maxwellnewage, Gilymiona, bakzkndd, mlobermeier, GabrielDornelas, and HighlandCuwu.
Bridge Shuffle
Create a function to bridge shuffle two arrays.
To bridge shuffle, you interleave the elements from both arrays in an alternating fashion:
array1 = ["A", "A", "A"]
array2 = ["B", "B", "B"]
shuffled_array = ["A", "B", "A", "B", "A", "B"]
This can still work with two arrays of uneven length. We simply tack on the extra elements from the longer array:
array1 = ["C", "C", "C", "C"]
array2 = ["D"]
shuffled_array = ["C", "D", "C", "C", "C"]
Examples
bridgeShuffle(["A", "A", "A"], ["B", "B", "B"])
output = ["A", "B", "A", "B", "A", "B"]
bridgeShuffle(["C", "C", "C", "C"], ["D"])
output = ["C", "D", "C", "C", "C"]
bridgeShuffle([1, 3, 5, 7], [2, 4, 6])
output = [1, 2, 3, 4, 5, 6, 7]
Notes
Elements in both arrays can be strings or integers.
If two arrays are of unequal length, add the additional elements of the longer array to the end of the shuffled array.
Always start your shuffle with the first element of Array 1.
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!
If you want to be mentioned here, I’d prefer if you sent a GitHub link or Replit!

Still working on those 2 videos… yes I’m slow
What did you expect?
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
What'd you think of today's email? |
Want to advertise in Sloth Bytes?
If your company is interested in reaching an audience of developers and programming enthusiasts, you may want to advertise with us here.
Reply