- Sloth Bytes
- Posts
- 🦥 How Do Files Work In The Cloud?
🦥 How Do Files Work In The Cloud?
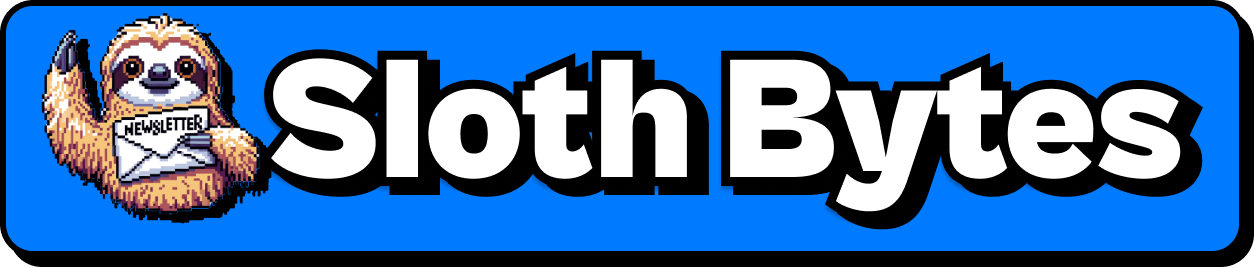
Hello friends!
Welcome to this week’s Sloth Bytes. I hope you had a great week!

Looking for unbiased, fact-based news? Join 1440 today.
Join over 4 million Americans who start their day with 1440 – your daily digest for unbiased, fact-centric news. From politics to sports, we cover it all by analyzing over 100 sources. Our concise, 5-minute read lands in your inbox each morning at no cost. Experience news without the noise; let 1440 help you make up your own mind. Sign up now and invite your friends and family to be part of the informed.

How Do Files Work In The Cloud?

You’ve probably uploaded files to Dropbox, Google Drive, or some other random website.
It works. It’s fast. It feels like magic.
But here’s the question: Where does that file actually go?
Let’s walk through how file storage in the cloud actually works.
It’s pretty interesting and good to know.
Step 1: You upload a file (or you think you did…)
You select a file. let’s say, sloth.jpg
and click upload.
Your browser doesn’t just teleport that file somewhere. Instead, it does this:
Breaks the file into binary data
Wraps it in an HTTP request
Sends that request to a cloud storage server
Usually via a POST request to a service like AWS S3, Google Cloud Storage, or a custom file-handling backend.
If you're using something like fetch()
or FormData
, this is what's happening under the hood.
<!-- Simple example of uploading a file with HTML forms -->
<form
action="http://fakeapi/api/upload"
method="POST"
enctype="multipart/form-data" <!-- This lets us upload files -->
>
<label for="image">Image</label>
<input type="file" name="image" id="image" />
<button type="submit">Submit</button>
</form>
What the backend would look like
Here’s a very simple Node.js backend to store a file.
import express from "express";
import multer from "multer";
const app = express();
const upload = multer({ dest: "uploads/" });
app.post("/upload", upload.single("image"), (req, res) => {
console.log(req.file); // info about the uploaded file
res.send("File uploaded!");
});
app.listen(3000, () => {
console.log("Server started on http://localhost:3000");
});
This file now lives in your backend’s file system or, more often, gets passed to a cloud storage provider.
Step 2: Storing the File in the Cloud (S3 Example)
The backend example doesn’t pass our image to a cloud provider.
Let’s change that.
Example of storing a file in the cloud (with S3)
// 🛠 Very simple example of uploading files to AWS S3
import { S3Client, PutObjectCommand } from "@aws-sdk/client-s3";
// Create an S3 client
const s3 = new S3Client({ region: "us-east-1" });
// Function to upload a file to S3
const uploadToS3 = async (fileBuffer, filename, mimetype) => {
// This object holds all the config details for the upload
const stuff_to_send = {
Bucket: "my-bucket",
// File path + name in the bucket
Key: `uploads/${filename}`,
// The actual file data in binary format
Body: fileBuffer,
// Helps S3 know how to serve the file (e.g. image/jpeg)
ContentType: mimetype
};
// Create the upload command with the config
const upload_file_command = new PutObjectCommand(stuff_to_send);
// Send the file to S3
const data = await s3.send(upload_file_command);
// Optional: log the response or return it
console.log("✅ File uploaded!", data);
};
Now in our backend example we just pass the file data to this uploadToS3 function:
import express from "express";
import multer from "multer"; // Helpful library for file uploads
import { uploadToS3 } from "./s3"; // Assuming you split logic
const upload = multer();
const app = express();
app.post("/upload", upload.single("image"), async (req, res) => {
try {
const { buffer, original_name, mimetype } = req.file;
await uploadToS3(buffer, original_name, mimetype);
res.send("✅ File uploaded to S3 successfully!");
} catch (error) {
console.error("Upload error:", error);
res.status(500).send("❌ Upload failed");
}
});
Note: If this code is wrong uhh, let me know please…
So what exactly is “the cloud” doing?
Once the image reaches the cloud, that file is:
Stored on a distributed storage system
Given a unique identifier or path (like
uploads/sloth-123.jpg
)Tagged with metadata like:
File type (
image/jpeg
)Size (say,
82 KB
)Permissions (who can access it)
Most cloud platforms also store copies of your file across multiple data centers for reliability, so if one server dies, your sloth pic still lives on 😌
This is called redundancy or replication, and it’s why cloud storage is way more resilient than your hard drive.
Bonus: Where does it actually live?
People love to say your file is “in the cloud”, but really, it's just sitting on someone else’s server.
That server might belong to:
Amazon - Amazon S3
Google - Google Cloud Storage
Microsoft - Azure Blob Storage
These systems use distributed architecture which means they store your file across multiple machines or locations, so it’s safe even if one server goes down.
They also use internal tools to keep track of where everything is, like a giant, super-organized file cabinet that spans the globe.
I wish I could explain those internal tools, but does it look like I’m smart enough to work there?
Uhhh what’s a blob?
“blob” just means Binary Large OBject, it’s a data type that stores unstructured binary data like like images, audio, video, or other non-textual files.
Step 3: Retrieving the File
When you (or someone else) wants to access sloth.jpg
, the browser sends a GET request like:
const imageUrl = `https://my-bucket.s3.amazonaws.com/uploads/sloth.jpg`;
For private files, you might generate a signed URL:
import { getSignedUrl } from "@aws-sdk/s3-request-presigner";
const url = await getSignedUrl(s3, new GetObjectCommand({
Bucket: "my-bucket",
Key: "uploads/sloth.jpg",
}), { expiresIn: 60 });
Now the user can access the file but only for the next 60 seconds.
There’s still a lot more about this…
If you’re curious and want to go more in-depth, check out these resources:
TL;DR
Your files in the cloud live on real servers, get split, tagged, copied, and served back to you through HTTP magic.
It might go through 3 data centers, 2 caches, a CDN, and a signed URL before showing up in your browser.
Cloud storage: not magic, just invisible infrastructure.


Thanks for the feedback :)



Thanks to everyone who submitted!
Unfortunately I yapped too much in this email and have a little bit of space before it gets cut off, so I can’t mention you all today sorry 😭
Sloth's Meal Time
Sloth is a very habitual person. He eats breakfast at 7:00 a.m. each morning, lunch at 12:00 p.m. and dinner at 7:00 p.m. in the evening.
Create a function that takes in the current time as a string and determines the duration of time before Sloth's next meal.
Represent this as an array with the first and second elements representing hours and minutes, respectively.
Examples
timeToEat("2:00 p.m.")
#5 hours until the next meal, dinner
output = [5, 0]
timeToEat("5:50 a.m.")
# 1 hour and 10 minutes until the next meal, breakfast
output = [1, 10]
How To Submit Answers
Reply with
A link to your solution (github, twitter, personal blog, portfolio, replit, etc)
or if you’re on the web version leave a comment!
If you want to be mentioned here, I’d prefer if you sent a GitHub link or Replit!

New videos coming out soon!
First video will be about AI agents and the second video will be about programming mistakes everyone makes.
That’s all from me!
Have a great week, be safe, make good choices, and have fun coding.
If I made a mistake or you have any questions, feel free to comment below or reply to the email!
See you all next week.
What'd you think of today's email? |
Want to advertise in Sloth Bytes?
If your company is interested in reaching an audience of developers and programming enthusiasts, you may want to advertise with us here.
Reply